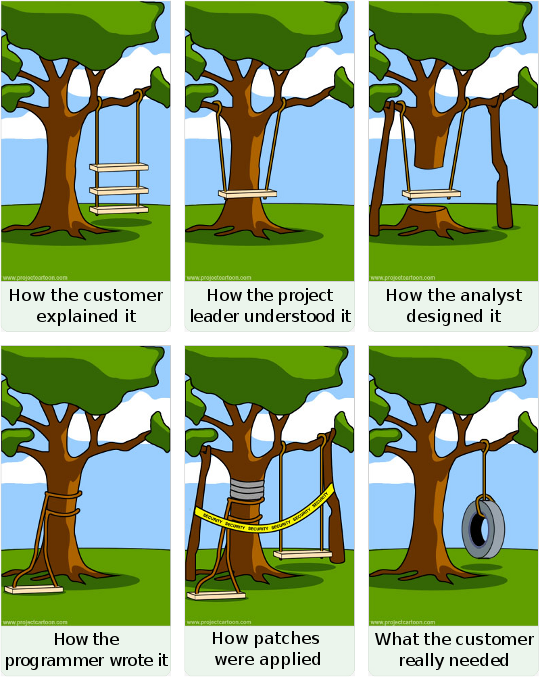
Funny isn’t it? But that is the way it goes in many companies developing software – especially in big companies and on big projects. This is the old school – but widely used – waterfall model. This software development process goes through the phases of Requirements, Design, Development, Testing & Maintenance one after the other. It relies on the dream that once a step is 100% complete, it is also 100% right and it drives us to the delivery of a working software even the one the customer wants. But it doesn’t go that way in the software industry: customers don’t know what they need and use to ask many useless features, project leaders don’t succeed to write down the perfect requirements, analysts spend ages building up software architectures writing down pages of diagrams – software is definitely one of the most complex artifact humans can build up – and developers are human too, so they make mistakes too.
The waterfall model is inspired by the industries, therefor by highly structured physical environments in which after-the-fact changes are prohibitively costly. We’re writing software and software are virtuals, right? So, you can change the whole architecture without any additional cost – except the time you spend updating the code. Enlarging an array is not as costly as enlarging a kitchen. Why do we use this development model then? Maybe because it is straightforward to build software like we would build an aircraft – or because people did not read correctly the article Winston W. Royce wrote in 1970 where it presents this model and said that it “is risky and invites failure”. :)
The solution: Agile methods!
It is easy and cheap to update software code. At least easier and cheaper than moving the kitchen upstairs. Therefor you can easily try & see. Try to implement a new feature and see if it 1) does work 2) is usefull. The rule of 80/20 applies very well in the software industry – for instance 80% of users use less than 20% of a software features. Since try & see looks like random programming I gonna present you some aspects of Agile development.
Many Agile development methods exist and they all follow the twelve principles of the Agile Manifesto. The four main values are:
Individuals and interactions over processes and tools
Working software over comprehensive documentation
Customer collaboration over contract negotiation
Responding to change over following a plan
Interactions, collaboration, embrace change… looks great isn’it?! But it’s easier to promote cool values than to follow them – and even harder to end up on a working software that suits user needs. To make it working, you have to follow an Agile method and work with people who want to follow it too.
Scrum — Get Agile!
Scrum) is an Agile development methods. It has been designed for management of software development projects but can be usedas a general project management approach.
Roles
Scrum defines three main roles in a Scrum team:
the Scrum Master, it maintains the process
the Product Owner, usually the customer ‑ it sets the directions of the software
the Team, it designs, develops, tests & deploys the software. The team is self-managed.
Scrum init
The scrum process is initialized with the creation of the product backlog. The product backlog can be seen as a wish list containing all the features the software should have. The Scrum team (including the Scrum master & the Product owner) is involved in the creation of the product backlog. The Product backlog is then reviewed and the features it contains are prioritized.
Three… Two… One… Ignition!
Once the product backlog is ready, the project is launched. The software development will be managed via small iterations from one to four weeks. At the end of each iteration, a new release of the software featuring new or updated features is deployed.
The following schema shows the iterative process:
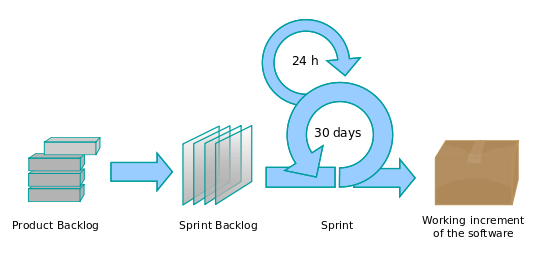
Each iterations (also called “sprint”) starts with the creation of a sprint backlog. The sprint backlog contains features coming from the product backlog which will be developed during the iteration. Everyday, a project status meeting called the Scrum occurs. The meeting is time-boxed to 15 minutes and everybody answers the three questions:
What have you done since yesterday?
What are you planning to do today?
Do you have any problem preventing you from accomplishing your goal?
At the end of each iteration, a new release of the software is deployed. The Product owner reviews this new release in order to validate it and to decide what the team has to developed or to refactor next.
The Scrum method does not describe the way developers code & test the application within a sprint allowing you to choose a method that suits your needs. The one I use is Behaviour Driven Development: it works as a charm with Scrum and it will be the topic of a future post.
I am Scrum master on a project involving four developers for the web agency O2Sources. We follow the Scrum method but we do not use every concepts and tools it offers like BurnDown chart#Burn_down) or Pig & Chicken roles#Roles). I didn’t talk about them in this post to keep it short and because I wanted to talk about concepts I know, I use and which works. Don’t hesitate to discover them by yourself – the wikipedia page of Scrum) could be a good start.
The project I am working on for O2Sources is called Townce. It is a Collaborative Working Web Application coded in Ruby on Rails. We follow the principles of Scrum & Behavior Driven Development methods to manage its development. Thanks to the Agile method Scrum, we deploy new releases every one to two weeks. Every release is reviewed to adjust the design in oder to make it more intuitive, to develop only essential features and to refactor the code. To see our baby evolve one week after the other could not be possible without the use of great methods and tools like Scrum, Behavior Driven Development, Ruby on Rails, Cucumber, RSpec… but these are other topics.